html・css・js(Vueをつかってみました)で、カレンダーのデイリー表示的なのを作ってみました。
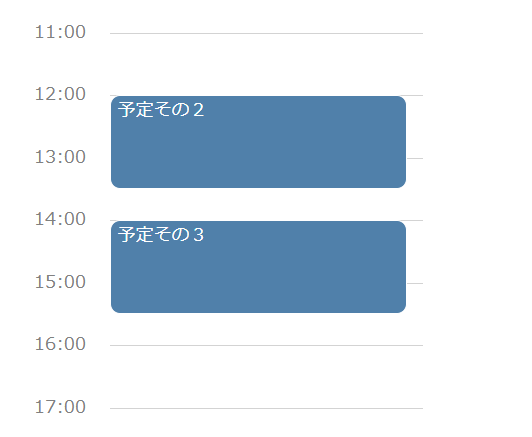
Vueのdataのeventsがイベント(タイトル、開始時刻、終了時刻)のオブジェクトを格納する配列になっています。
<!DOCTYPE html> <html lang="ja"> <head> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>schedule</title> <style> #daily-calendar * { box-sizing: border-box; } .time-cell { width: 80px; height: 50px; line-height: 50px; text-align: center; color: grey; font-size: 90%; } .event-bg-cell { width: 250px; height: 50px; border-top: solid lightgray 1px; } .event-bg-cell:last-child { border-bottom: solid lightgray 1px; } .event { position: absolute; width: 95%; padding-left: 5px; border: solid 1px white; border-radius: 8px; top: 0px; font-size: 90%; color: white; background-color:#5080AA; } </style> </head> <body> <div id="daily-calendar" style="display: flex; width: 330px;" > <div> <div v-for="n in 25" class="time-cell"> {{ (n - 1) + ":00" }} </div> </div> <div style="position: relative; margin: 25px 0;"> <div> <div v-for="n in 24" class="event-bg-cell"></div> </div> <div v-for="event in events" :style="{ top: getPosition(event.dtFrom) + 'px', height: getPosition(event.dtTo) - getPosition(event.dtFrom) + 'px' }" class="event" > {{ event.title }} </div> </div> </div> <script src="https://unpkg.com/vue@next"></script> <script> Vue.createApp({ data() { return { events: [ { title: "予定その1", dtFrom: new Date(2021, 8-1, 26, 8, 00), dtTo: new Date(2021, 8-1, 26, 10, 30) }, { title: "予定その2", dtFrom: new Date(2021, 8-1, 26, 12, 00), dtTo: new Date(2021, 8-1, 26, 13, 30) }, { title: "予定その3", dtFrom: new Date(2021, 8-1, 26, 14, 00), dtTo: new Date(2021, 8-1, 26, 15, 30) } ] } }, methods: { // 時刻からスケジュールのY位置を算出する getPosition: function(dateTime){ const now = new Date() // 本日00:00のDateオブジェクト const todaysStart = new Date() todaysStart.setHours(0, 0, 0, 0) // 本日24:00のDateオブジェクト const todaysEnd = new Date() todaysEnd.setDate(todaysEnd.getDate() + 1) todaysEnd.setHours(0, 0, 0, 0) // カレンダー最上部からの位置(px)を返す if ( dateTime <= todaysStart ) { return 0 // 本日00:00以前の場合は最上部 } else if ( dateTime >= todaysEnd ) { return 50 * 24 // 本日24:00以降の場合は最下部 } else { // 本日00:00からの時間数 * 50px const hourFromStart = ( dateTime.getHours() + (dateTime.getMinutes() / 60) ) return hourFromStart * 50 } } } }).mount('#daily-calendar') </script> </body> </html>
コメント